# 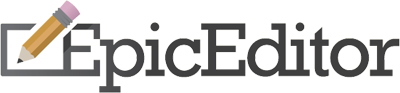
## An Embeddable JavaScript Markdown Editor
EpicEditor is an embeddable JavaScript [Markdown](http://daringfireball.net/projects/markdown/) editor with split fullscreen editing, live previewing, automatic draft saving, offline support, and more. For developers, it offers a robust API, can be easily themed, and allows you to swap out the bundled Markdown parser with anything you throw at it.
## Why
Because, WYSIWYGs suck. Markdown is quickly becoming the replacement. [GitHub](http://github.com), [Stackoverflow](http://stackoverflow.com), and even blogging apps like [Posterous](http://posterous.com) are now supporting Markdown. EpicEditor allows you to create a Markdown editor with a single line of JavaScript:
```javascript
var editor = new EpicEditor().load();
```
## Quick Start
EpicEditor is easy to implement. Add the script and assets to your page, provide a target container and call `load()`.
### Step 1: Download
[Download the latest release](http://epiceditor.com) or clone the repo:
```bash
$ git clone git@github.com:OscarGodson/EpicEditor
```
### Step 2: Create your container element
```html
```
### Step 3: Add the `epiceditor.js` file
```html
```
### Step 4: Init EpicEditor
```javascript
var editor = new EpicEditor().load();
```
## API
### EpicEditor([_options_])
The `EpicEditor` constructor creates a new editor instance. Customize the instance by passing the `options` parameter. The example below uses all options and their defaults:
```javascript
var opts = {
container: 'epiceditor',
basePath: 'epiceditor',
localStorageName: 'epiceditor',
parser: marked,
file: {
name: 'epiceditor',
defaultContent: '',
autoSave: 100
},
theme: {
base:'/themes/base/epiceditor.css',
preview:'/themes/preview/preview-dark.css',
editor:'/themes/editor/epic-dark.css'
},
focusOnLoad: false,
shortcut: {
modifier: 18,
fullscreen: 70,
preview: 80,
edit: 79
}
}
var editor = new EpicEditor(opts);
```
### Options
Option |
Description |
Default |
`container` |
The ID (string) or element (object) of the target container in which you want the editor to appear. |
`epiceditor` |
`basePath` |
The base path of the directory containing the `/themes`, `/images`, etc. |
`epiceditor` |
`localStorageName` |
The name to use for the localStorage object. |
`epiceditor` |
`parser` |
[Marked](https://github.com/chjj/marked) is the only parser built into EpicEditor, but you can customize or toggle this by passing a parsing function to this option. For example:
parser: MyCustomParser.parse |
`marked` |
`focusOnLoad` |
If `true`, editor will focus on load. |
`false` |
`file.name` |
If no file exists with this name a new one will be made, otherwise the existing will be opened. |
container ID |
`file.defaultContent` |
The content to show if no content exists for a file. |
|
`file.autoSave` |
How often to auto save the file in milliseconds. Set to `false` to turn it off. |
`100` |
`theme.base` |
The base styles such as the utility bar with the buttons. |
`themes/base/epiceditor.css` |
`theme.editor` |
The theme for the editor which is the area you type into. |
`themes/editor/epic-dark.css` |
`theme.preview` |
The theme for the previewer. |
`themes/preview/github.css` |
`shortcut.modifier` |
The key to hold while holding the other shortcut keys to trigger a key combo. |
`18` (`alt` key) |
`shortcut.fullscreen` |
The shortcut to open fullscreen. |
`70` (`f` key) |
`shortcut.preview` |
The shortcut to open the previewer. |
`80` (`p` key) |
`shortcut.edit` |
The shortcut to open the editor. |
`79` (`o` key) |
### load([_callback_])
Loads the editor by inserting it into the DOM by creating an `iframe`. Will trigger the `load` event, or you can provide a callback.
```javascript
editor.load(function () { console.log("Editor loaded.") });
```
### unload([_callback_])
Unloads the editor by removing the `iframe`. Keeps any options and file contents so you can easily call `.load()` again. Will trigger the `unload` event, or you can provide a callback.
```javascript
editor.unload(function () { console.log("Editor unloaded.") });
```
### getElement(_element_)
Grabs an editor element for easy DOM manipulation. See the Themes section below for more on the layout of EpicEditor elements.
* `container`: The element given at setup in the options.
* `wrapper`: The wrapping `` containing the 2 editor and previewer iframes.
* `wrapperIframe`: The iframe containing the `wrapper` element.
* `editor`: The #document of the editor iframe (i.e. you could do `editor.getElement('editor').body`).
* `editorIframe`: The iframe containing the `editor` element.
* `previewer`: The #document of the previewer iframe (i.e. you could do `editor.getElement('previewer').body`).
* `previewerIframe`: The iframe containing the `previewer` element.
```javascript
someBtn.onclick = function(){
console.log(editor.getElement('editor').body.innerHTML); // Returns the editor's content
}
```
### open(_filename_)
Opens a file into the editor.
```javascript
openFileBtn.onclick = function(){
editor.open('some-file'); // Opens a file when the user clicks this button
}
```
### importFile([_filename_],[_content_])
Imports a string of content into a file. If the file already exists, it will be overwritten. Useful if you want to inject a bunch of content via AJAX. Will also run `.open()` after import automatically.
```javascript
importFileBtn.onclick = function(){
editor.importFile('some-file',"#Imported markdown\nFancy, huh?"); //Imports a file when the user clicks this button
}
```
### exportFile([_filename_],[_type_])
Returns the raw content of the file by default, or if given a `type` will return the content converted into that type. If you leave both parameters `null` it will return the current document's raw content.
```javascript
syncWithServerBtn.onclick = function(){
var theContent = editor.exportFile();
saveToServerAjaxCall('/save', {data:theContent}, function () {
console.log('Data was saved to the database.');
});
}
```
### rename(_oldName_, _newName_)
Renames a file.
```javascript
renameFileBtn.onclick = function(){
var newName = prompt('What do you want to rename this file to?');
editor.rename('old-filename.md', newName); //Prompts a user and renames a file on button click
}
```
### save()
Manually saves a file. EpicEditor will save continuously every 100ms by default, but if you set `autoSave` in the options to `false` or to longer intervals it's useful to manually save.
```javascript
saveFileBtn.onclick = function(){
editor.save();
}
```
### remove(_name_)
Deletes a file.
```javascript
removeFileBtn.onclick = function(){
editor.remove('example.md');
}
```
### on(_event_, _handler_)
Sets up an event handler (callback) for a specified event. For all event types, see the Events section below.
```javascript
editor.on('unload',function(){
console.log('Editor was removed');
});
```
### emit(_event_)
Fires an event programatically. Similar to jQuery's `.trigger()`
```javascript
editor.emit('unload'); // Triggers the handler provided in the "on" method above
```
### removeListener(_event_, [_handler_])
Allows you to remove all listeners for an event, or just the specified one.
```javascript
editor.removeListener('unload'); //The handler above would no longer fire
```
### preview()
Puts the editor into preview mode.
```javascript
previewBtn.onclick = function(){
editor.preview();
}
```
### edit()
Puts the editor into edit mode.
```javascript
editBtn.onclick = function () {
editor.edit();
}
```
## Events
You can hook into specific events in EpicEditor with
on()
such as when a file is
created, removed, or updated. Below is a complete list of currently supported events and their description.
Event Name |
Description |
`create` |
Fires whenever a new file is created. |
`read` |
Fires whenever a file is read. |
`update` |
Fires whenever a file is updated. |
`remove` |
Fires whenever a file is deleted. |
`load` |
Fires when the editor loads via `load()`. |
`unload` |
Fires whenever the editor is unloaded via `unload()` |
`preview` |
Fires whenever the previewer is opened (excluding fullscreen) via `preview()` or the preview button. |
`edit` |
Fires whenever the editor is opened (excluding fullscreen) via `edit()` or the edit button. |
`save` |
Fires whenever the file is saved whether by EpicEditor automatically or when `save()` is called. |
`open` |
Fires whenever a file is opened or loads automatically by EpicEditor or when `open()` is called. |
## Themes
Theming is easy in EpicEditor. There are three different `